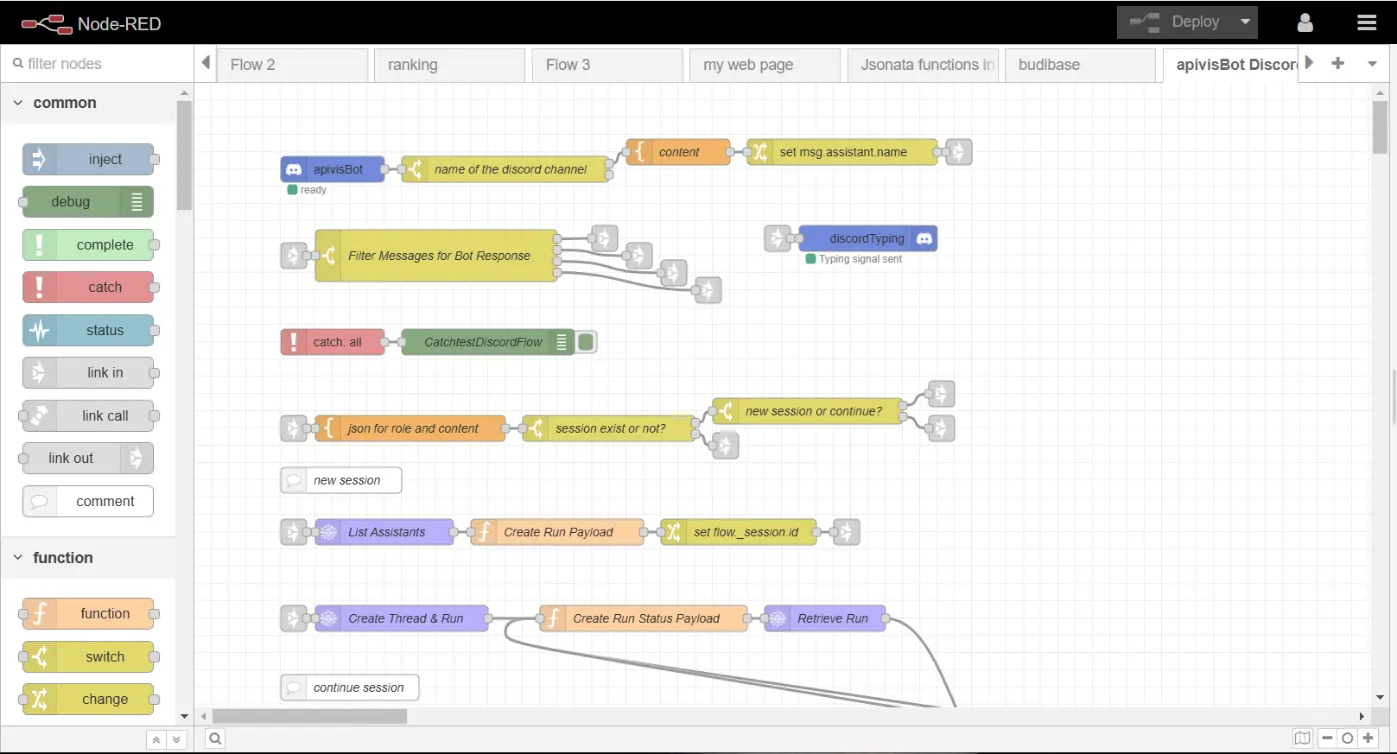
In this post, we'll walk you through how to create a smart and interactive Discord bot using Node-RED and OpenAI. Our bot listens to user commands, processes them with OpenAI's powerful language model, and responds intelligently in your Discord channel. Let's dive into how all of this comes together!
Overview
Our flow is designed to accomplish these tasks:
• Listen to messages in a specific Discord channel (e.g., "support").
• Identify when users are addressing the bot.
• Manage conversations intelligently, keeping track of new and ongoing sessions.
• Use OpenAI to generate responses to user queries.
• Handle different statuses of the conversation to ensure smooth interaction.
Nodes Breakdown
Here's a peek into the main components of our Node-RED flow:
1.) Listening to Discord Messages:
• (apivisBot): This node picks up every message in the Discord server. It’s our bot's ears in the support channel.
2.) Filtering Relevant Messages:
• switch (name of the discord channel): This node verifies that messages are from the 'support' channel.
• switch (Filter Messages for Bot Response): This smart filter ensures only messages directed at the bot (e.g., containing "Hey Bot") are processed further. In this flow all messages are passed on with the otherwise filter. Remove that one so that the bot is not answer all conversations.
3.) Processing Messages:
• template (json for role and content): Prepares the message content for our AI assistant by creating a JSON structure.
• change nodes: Assist in setting necessary fields and managing session IDs.
• OpenAI API Integration Nodes:
listAssistants: Fetches available assistants from OpenAI.
createThreadAndRun: Initiates a conversation thread with OpenAI.
createMessage: Sends the user’s message to OpenAI.
retrieveRun: Checks the status of the conversation.
4.) Handling Conversations:
• switch (session exist or not?) and switch (new session or continue?): These nodes manage whether the conversation is a new one or an ongoing session.
• function nodes: These craft the necessary payloads for each step and manage conversation threads.
5.) Responding to Users:
• discordMessageManager: Sends OpenAI’s responses back to the user in Discord.
•discordTyping: Simulates typing status to let users know the bot is processing their request.
How It All Connects
1.) Initialization:
• The flow starts when a message is detected in the Discord 'support' channel.
• The switch nodes filter out irrelevant messages and ensure only commands for the bot are processed.
2.) Session Management:
• If it’s a brand-new conversation, the bot kicks off a new session with OpenAI using the createThreadAndRun node.
• For ongoing conversations, the bot retrieves the existing thread and adds to it. This is managed by the switch (session exist or not?) node.
3.) Creating and Sending Messages:
• The template node structures the user’s input, and createMessage sends it to OpenAI.
• The status of this message is tracked using retrieveRun, and based on the status (queued, in progress, completed), appropriate actions are taken.
4.) Generating Responses:
• OpenAI processes the input, and the bot fetches the response with listMessages.
• To handle Discord's message limits, messages longer than 2000 characters are split and sent in chunks by the splitLongMessages function.
5.) User Feedback:
• Finally, discordMessageManager ensures the bot’s response reaches the user, and discordTyping simulates real-time typing, enhancing the user experience.
How to setup Discord
To start using Discord I found the following site very helpful: https://www.writebots.com